Table of Contents
How to Implement Recursion in Python-The Complete Guide
Recursion in Python is one of the most powerful concepts in programming that helps break a complex problem into a few simpler ones. In Python, this allows a function to call itself, reducing redundancy and improving readability. Further in this article, we will learn how to implement recursion in Python, how it works, and some best practices for optimizing it.
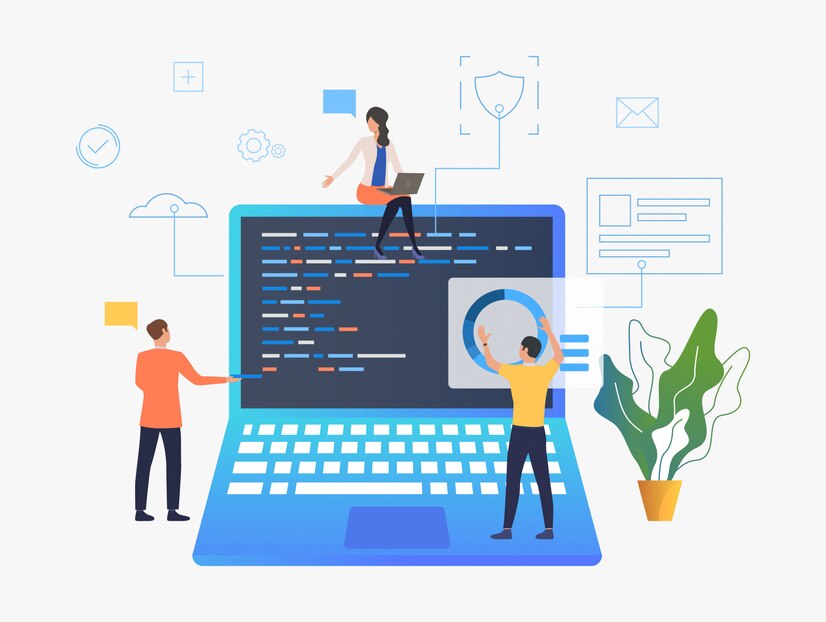
What is Recursion in Python?
Recursion in Python when a function calls itself from within. Each time one of the recursive calls is executed, the recursive function processes a smaller problem until that function hits the base case, then stops calling itself.
- Why Use Recursion?
- It is used in some divide-and-conquer algorithms, which make them easier to solve.
- Fewer lines of code for a problem that may be quite complex.
Improved readability for mathematical computations, such as when calculating the factorial or Fibonacci series.
How to Implement Recursion in Python
The implementation of recursion, in general, can be achieved very effectively by following the steps as follows:
1. Define a Base Case.
A base case is what conditions apply for the recursion to stop. If you fail to define a base case, then your function will call itself repeatedly into an infinite loop that could lead to a stack overflow.
2. The Recursive Case
The recursive case is where the function calls itself with a smaller version of the original problem until it reaches a base case.
Example 1: Computing Factorials Using Recursion in Python
def factorial(n):
if n == 0:
return 1 # Base case
return n * factorial(n - 1) # Recursive case
print(factorial(5)) # Output: 120
Understanding Recursive Function Execution
When calling factorial(5), Python follows these steps:
- factorial(5) calls factorial(4)
- factorial(4) calls factorial(3)
- factorial(3) calls factorial(2)
- factorial(2) calls factorial(1)
- factorial(1) calls factorial(0), which returns 1 (base case)
- The results propagate back, calculating 5 * 4 * 3 * 2 * 1 = 120
Example 2: Fibonacci Series Using Recursion
def fibonacci(n):
if n <= 1:
return n # Base case
return fibonacci(n - 1) + fibonacci(n - 2) # Recursive case
print(fibonacci(6)) # Output: 8
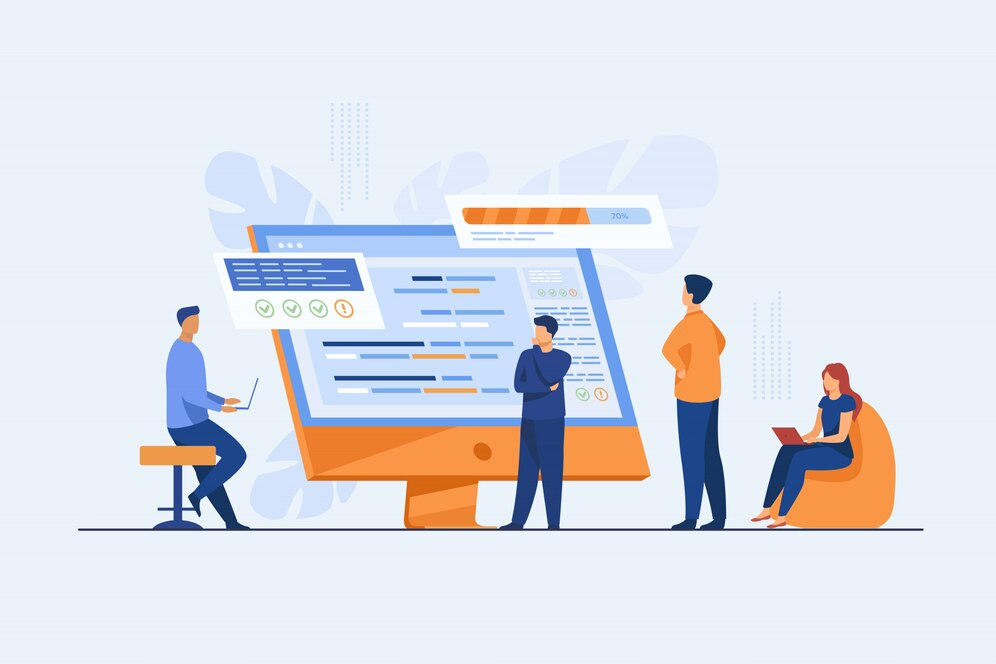
Optimizing Recursive Functions
Using recursion without optimization can lead to stack overflow errors or inefficient computations. Here are ways to improve performance:
1. Memoization (Caching Results)
Memorization stores previously computed results to avoid redundant calculations.
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n - 1) + fibonacci(n - 2)
print(fibonacci(50)) # Output: 12586269025
2. Tail Recursion Optimization
Tail recursion eliminates extra function calls stored in memory, improving efficiency.
def tail_factorial(n, acc=1):
if n == 0:
return acc
return tail_factorial(n - 1, acc * n)
print(tail_factorial(5)) # Output: 120
When to Use Recursion vs. Iteration
While recursion simplifies problems, it’s not always the best choice. Use recursion when:
- The problem has a natural recursive structure (e.g., tree traversal, graph search).
- The function can be optimized using memoization or tail recursion.
For large datasets, iteration is often more memory-efficient than recursion.
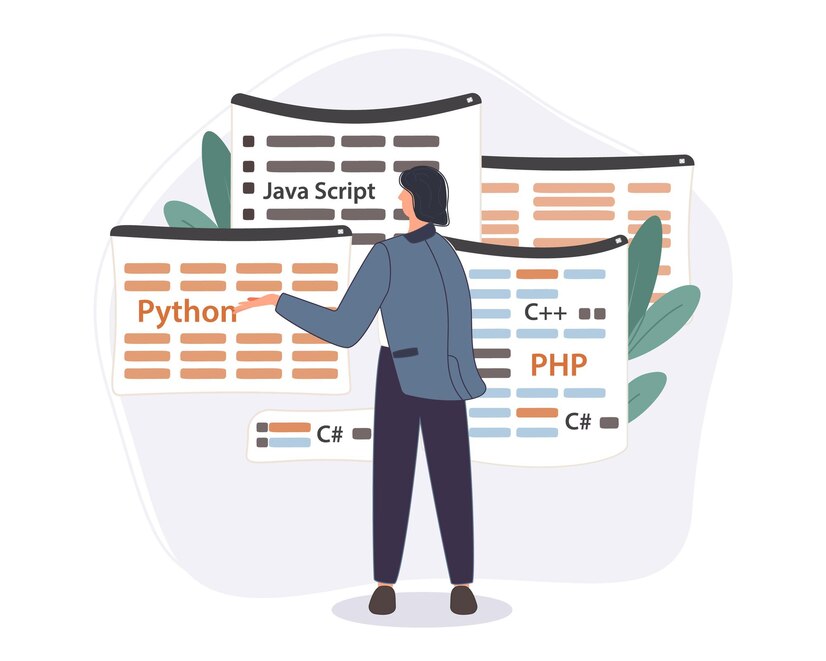
Common Errors in Recursion and How to Fix Them
1.Infinite Recursion: Forgetting the base case leads to infinite function calls.
- Fix: Ensure a proper stopping condition.
2. Excessive Memory Usage: Too many recursive calls consume stack space.
- Fix: Use iteration, memoization, or tail recursion.
3. Performance Issues: Recursion without optimization slows down execution.
- Fix: Implement caching or convert to iteration.
Conclusion
Learning how to implement recursion in Python is crucial for problem-solving in programming. By defining clear base cases, writing efficient recursive cases, and applying optimization techniques, you can make recursion both powerful and practical. Whether you’re solving factorial problems, Fibonacci series, or tree traversals, mastering recursion enhances your coding skills.
One Team Solutions is one of the Best Software Training Institutes in Kochi, Kerala. One Team Offers Python Course in Trivandrum, PHP Training, Dot Net Training, Node Training, React Training, IOS/Android Training & Digital Marketing Course in Trivandrum & Angularjs course in Kochi for Freshers and Experienced Professionals.
The Training Team of One Team is well experienced and the best in the Industry. And we often conduct activities to prepare for GD (Group Discussion) and JAM (Just a Minute).
We conduct mock interviews and will discuss the positives and negatives with students. Our daily aptitude tests will improve the student’s ability to attend those tests in interviews.
Stepping stones to a successful career can be the some certifications for Python programming, Cloud Computing, and AI. Take the first step today to secure your future in the dynamic IT market of Kerala.